目次
コントローラーの設定
コントローラー
package com.example.xxx;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
@RestController
public class XXXController {
@GetMapping("/file/download")
public ResponseEntity<Resource> downloadFile() {
Path path = Paths.get("image/test.jpg");
Resource resource;
try {
resource = new UrlResource(path.toUri());
} catch (Exception e) {
throw new RuntimeException("Issue reading the file.", e);
}
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
@Operation(summary = "Upload a file")
@PostMapping(value = "/file/upload", consumes = MediaType.MULTIPART_FORM_DATA_VALUE)
public ResponseEntity<String> uploadFile(@Parameter(description = "File to be uploaded", required = true,
content = @Content(mediaType = MediaType.MULTIPART_FORM_DATA_VALUE,
schema = @Schema(type = "string", format = "binary")))
MultipartFile file) {
if (file.isEmpty()) {
return ResponseEntity.badRequest().body("Cannot upload empty file");
}
try {
byte[] bytes = file.getBytes();
Path path = Paths.get("image/upload/" + file.getOriginalFilename());
Files.write(path, bytes);
} catch (Exception e) {
return ResponseEntity.status(500).body("Failed to upload the file");
}
return ResponseEntity.ok().body("File successfully uploaded");
}
}
アノテーションの説明
以下がアノテーションの説明です。
@Operation(summary = "Upload a file")
:- このアノテーションは、Swagger UIやその他のAPIドキュメンテーションツールにエンドポイントの説明を提供するためのものです。この場合、エンドポイントの要約として “Upload a file” が表示されます。
@PostMapping(value = "/file/upload", consumes = MediaType.MULTIPART_FORM_DATA_VALUE)
:
- Spring Bootのエンドポイントを定義するためのアノテーションです。このエンドポイントは、POSTリクエストを待ち受けており、
/file/upload
というURLでアクセス可能です。 consumes = MediaType.MULTIPART_FORM_DATA_VALUE
は、このエンドポイントがmultipart/form-data
のメディアタイプのデータを受け入れることを意味します。これは、ファイルのアップロードに使用される一般的なコンテンツタイプです。@Parameter
アノテーション:- このアノテーションは、エンドポイントのパラメータに関する詳細情報を提供します。
description = "File to be uploaded"
: パラメータの説明です。required = true
: このパラメータは必須であることを示します。content = @Content(...)
: ここでは、パラメータのコンテンツタイプやスキーマに関する詳細情報を提供します。mediaType = MediaType.MULTIPART_FORM_DATA_VALUE
: パラメータがmultipart/form-data
のメディアタイプを持つことを示します。schema = @Schema(type = "string", format = "binary")
: このスキーマ定義は、パラメータがバイナリフォーマットの文字列であることを示しています(この場合、ファイル)。
MultipartFile file
:- これは、Spring Bootが提供する
MultipartFile
型を使用して、アップロードされたファイルを受け取るための引数です。
要するに、このエンドポイントは、ユーザーがファイルをアップロードすることを許可し、アップロードされたファイルを処理するためのものです。また、Swagger UIでのドキュメンテーションに関する詳細情報も提供されています。
Swaggerでファイルのダウンロードとアップロードの検証
実際にSwaggerで検証してみます。
ダウンロード
Try It Out > Execute > Donload fileでファイルがダウンロードされます。
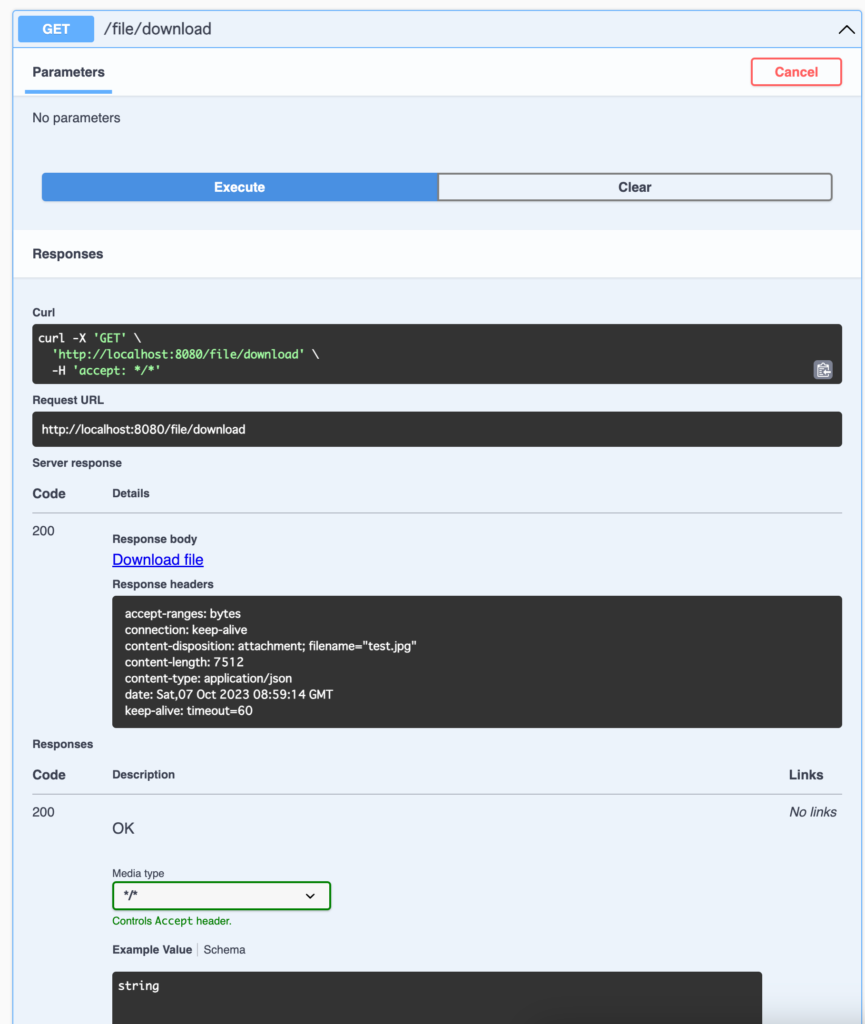
アップロード
Try It Out > Choose Fileで適当なファイルを選択 > Execute でファイルがアップロードされ、アプリケーションの所定のディレクトリに保存されます。
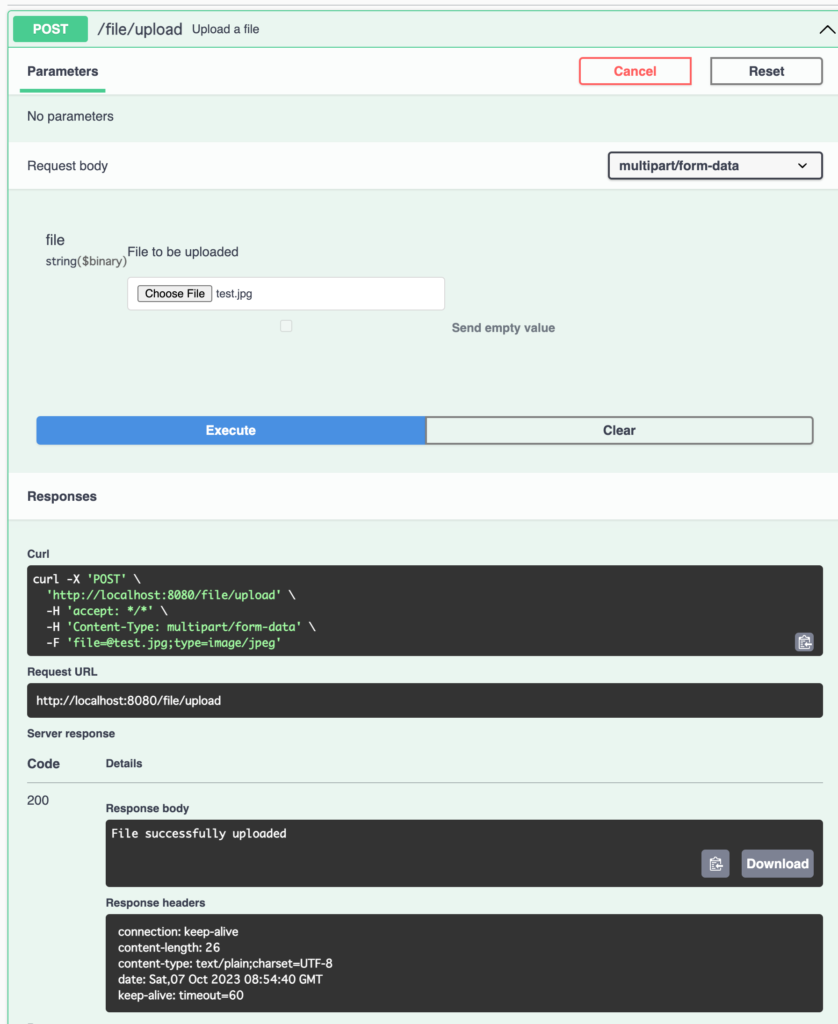