Mavenリポジトリの種類
Mavenのリポジトリは以下のように分類されます.
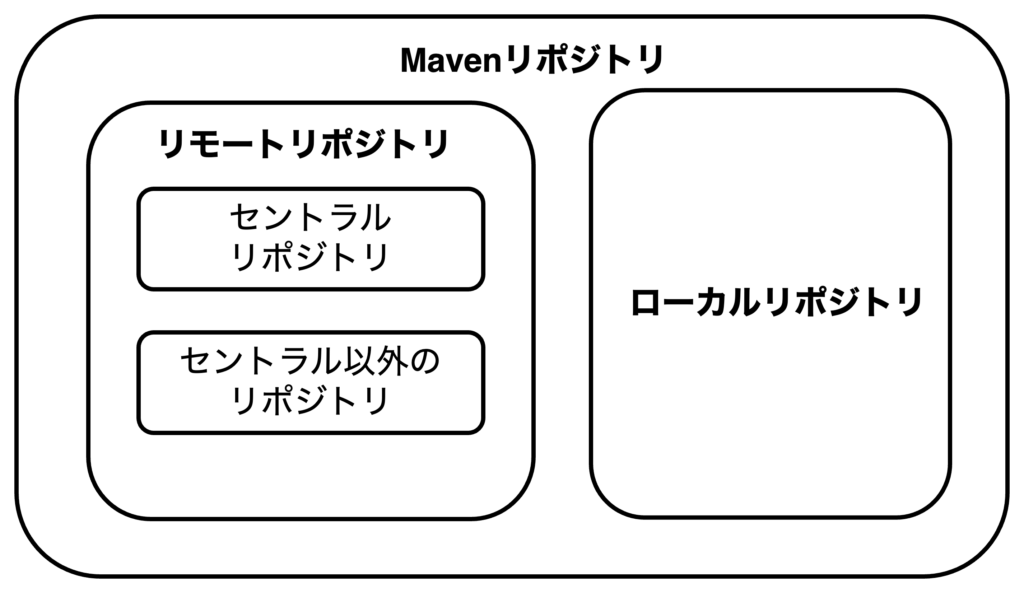
- リモートリポジトリ:公開されているリポジトリ
- セントラルリポジトリ:Mavenによって管理されているリポジトリ
- セントラル以外のリポジトリ:Maven以外の組織で管理されているリポジトリ
- ローカルリポジトリ:自身の組織内で利用する非公開のリポジトリ
リモートリポジトリ(セントラルリポジトリ)の利用
セントラルリポジトリの情報は暗黙で定義されています.そのため,セントラルリポジトリを利用するのに特別な手順は不要です.従来どおり以下のように行うことでライブラリを利用できます.
- ライブラリを利用したいプロジェクトをMaven Central Repository Searchで検索
- ライブラリのスニペットをpom.xmlのdependenciesまたはbuildsに追加
リモートリポジトリ(セントラル以外)の利用
セントラル以外のリポジトリを利用する場合は,リポジトリの情報をpom.xmlに記載する必要があります.ここでは例としてSpringのAspectJを利用します.
ライブラリを利用するプロジェクトを作成します
% mvn archetype:generate
プロジェクトの情報として以下を設定します.
groupId: org.example
artifactId: repository-sample
version: 1.0-SNAPSHOT
packaging: jar
name: repository-sample
pom.xmlを編集します
編集のポイントは3点です.
- spring.orgのリポジトリを追加
<repositories>
<repository>
<id>spring.org</id>
<name>spring foundation repository</name>
<url>http://repo.spring.io/release/</url>
</repository>
</repositories>
- aspectjのdependencyを追加
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.9.19</version>
</dependency>
- exec:javaを実行できるようにexec-maven-pluginをbuildに追加
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>3.1.0</version>
<configuration>
<mainClass>org.example.App</mainClass>
</configuration>
</plugin>
pom.xmlの全体はこちらです.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>repository-sample</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<name>repository-sample</name>
<!-- FIXME change it to the project's website -->
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
<repositories>
<repository>
<id>spring.org</id>
<name>spring foundation repository</name>
<url>http://repo.spring.io/release/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.9.19</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>3.1.0</version>
<configuration>
<mainClass>org.example.App</mainClass>
</configuration>
</plugin>
</plugins>
<pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) -->
<plugins>
<!-- clean lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#clean_Lifecycle -->
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
</plugin>
<!-- default lifecycle, jar packaging: see https://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_jar_packaging -->
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.1</version>
</plugin>
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-install-plugin</artifactId>
<version>2.5.2</version>
</plugin>
<plugin>
<artifactId>maven-deploy-plugin</artifactId>
<version>2.8.2</version>
</plugin>
<!-- site lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#site_Lifecycle -->
<plugin>
<artifactId>maven-site-plugin</artifactId>
<version>3.7.1</version>
</plugin>
<plugin>
<artifactId>maven-project-info-reports-plugin</artifactId>
<version>3.0.0</version>
</plugin>
</plugins>
</pluginManagement>
</build>
</project>
プロジェクトのパッケージ化
mvn package
します.問題なくpackageゴールが完了します.
% mvn package
以上がセントラルリポジトリ以外のリモートリポジトリを使う方法です.
ローカルプロジェクトの利用
次にローカルプロジェクトの利用です.これは自身で作成したプロジェクトをjarとしてライブラリ化したものを,他のプロジェクトで使いたいといったケースが該当します.
ライブラリ用プロジェクトの作成
% mvn archetype:generate
プロジェクトの情報として以下を設定します.
groupId: org.example.lib
artifactId: mvn-lib
version: 1.0
packaging: jar
name: my-maven-library
pom.xmlを編集します.exec:javaが利用できるようにbuildにexec-maven-plugin
を追加します.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example.lib</groupId>
<artifactId>mvn-lib</artifactId>
<version>1.0</version>
<packaging>jar</packaging>
<name>my-maven-library</name>
<!-- FIXME change it to the project's website -->
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.5.0</version>
<configuration>
<mainClass>org.example.lib.App</mainClass>
</configuration>
</plugin>
</plugins>
<pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) -->
<plugins>
<!-- clean lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#clean_Lifecycle -->
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
</plugin>
<!-- default lifecycle, jar packaging: see https://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_jar_packaging -->
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.1</version>
</plugin>
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-install-plugin</artifactId>
<version>2.5.2</version>
</plugin>
<plugin>
<artifactId>maven-deploy-plugin</artifactId>
<version>2.8.2</version>
</plugin>
<!-- site lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#site_Lifecycle -->
<plugin>
<artifactId>maven-site-plugin</artifactId>
<version>3.7.1</version>
</plugin>
<plugin>
<artifactId>maven-project-info-reports-plugin</artifactId>
<version>3.0.0</version>
</plugin>
</plugins>
</pluginManagement>
</build>
</project>
App.javaを編集します
package org.example.lib;
public class App {
public static void main(String[] args) {
System.out.println(new Lib("Hanako", 29));
}
}
AppTest.javaにも簡単なテストを書いておきます.
package org.example.lib;
import static org.junit.Assert.assertTrue;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.junit.Test;
public class AppTest extends TestCase
{
public AppTest(String testName) {
super(testName);
}
public void testApp() {
App app = new App();
assertNotNull(app);
}
public void testLib() {
Lib lib = new Lib("hoge", 29);
assertNotNull(lib);
}
@Test
public void shouldAnswerWithTrue()
{
assertTrue( true );
}
}
実行します.
% mvn clean package
% mvn exec:java
以下のようにメッセージが表示されたら成功です.
Hello, I am Hanako. I am 29 years old.
完成したライブラリをローカルリポジトリに登録します.ライブラリのプロジェクトmvn-lib
直下から以下を実行します.
% mvn install
これでライブラリがローカルプロジェクトに登録されました.既に用意されているjarファイルがある場合,以下のコマンドでローカルリポジトリに追加することも可能です.
% mvn install:install-file
-Dfile=ライブラリファイルのパス (.../mvn-lib-1.0.jar)
-DgroupId=グループID (org.example.lib)
-DartifactId=アーティファクトID (mvn-lib)
-Dpackaging=パッケージング (jar)
-Dversion=バージョン (1.0)
ライブラリプロジェクトの利用
続いて,作成したライブラリを他のプロジェクトで利用します.ここでは「リモートリポジトリ(セントラル以外)の利用」で作成したrepository-sample
で利用します.
pom.xmlに作成したライブラリをdependency配下に追加します.
<dependency>
<groupId>org.example.lib</groupId>
<artifactId>mvn-lib</artifactId>
<version>1.0</version>
</dependency>
最終的なpom.xmlは以下のようになります.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>repository-sample</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<name>repository-sample</name>
<!-- FIXME change it to the project's website -->
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
<repositories>
<repository>
<id>spring.org</id>
<name>spring foundation repository</name>
<url>http://repo.spring.io/release/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.9.19</version>
</dependency>
<dependency>
<groupId>org.example.lib</groupId>
<artifactId>mvn-lib</artifactId>
<version>1.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>3.1.0</version>
<configuration>
<mainClass>org.example.App</mainClass>
</configuration>
</plugin>
</plugins>
<pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) -->
<plugins>
<!-- clean lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#clean_Lifecycle -->
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
</plugin>
<!-- default lifecycle, jar packaging: see https://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_jar_packaging -->
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.1</version>
</plugin>
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-install-plugin</artifactId>
<version>2.5.2</version>
</plugin>
<plugin>
<artifactId>maven-deploy-plugin</artifactId>
<version>2.8.2</version>
</plugin>
<!-- site lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#site_Lifecycle -->
<plugin>
<artifactId>maven-site-plugin</artifactId>
<version>3.7.1</version>
</plugin>
<plugin>
<artifactId>maven-project-info-reports-plugin</artifactId>
<version>3.0.0</version>
</plugin>
</plugins>
</pluginManagement>
</build>
</project>
App.javaを編集します.
package org.example;
import org.example.lib.Lib;
public class App
{
public static void main( String[] args )
{
Lib lib = new Lib("Satoru", 32);
System.out.println(lib.greeting());
}
}
実行します.
% mvn clean package
% mvn exec:java
以下のようにメッセージが出力されたら成功です.
Hello, I am Satoru. I am 32 years old.